Web scraping is a powerful technique used to gather data from websites automatically. It bypasses the need for manual extraction. This process involves programmatically accessing web pages and extracting useful information. It’s used for various tasks such as market research, price comparison, and lead generation.
Selenium, primarily a browser automation tool, is also popular for web scraping. It can mimic user interactions with web pages, making it ideal for extracting data from dynamically generated content.
Selenium manages cookies, sessions, and pop-ups, making it essential for complex scraping tasks that go beyond basic HTTP requests. This guide will walk you through using Selenium for web scraping, ensuring you have the know-how to tackle projects that require sophisticated data extraction.
Setting Up Your Environment
To start using Selenium for web scraping, you’ll first need to set up your development environment. This setup involves two main steps:
1. Installing Selenium and WebDriver:
Begin by installing the Selenium package. If you are using Python, you can install it via pip with the command: pip install selenium.
You will also need a WebDriver for the browser you plan to automate. For example, Chrome users can download Chromedriver, and Firefox users can download Geckodriver. Ensure the driver is placed in a location accessible to your system’s PATH or specify the path in your code.
2. Configuring Your Development Environment:
Once Selenium and the appropriate WebDriver are installed, configure your IDE (Integrated Development Environment) to use Selenium. This might involve setting up project folders for your scripts and ensuring your environment recognizes the Selenium library.
Optionally, you can set up virtual environments to manage dependencies and versions specific to your scraping projects, using virtualenv or conda.
This setup will prepare your system for running Selenium scripts that interact with web browsers to perform tasks ranging from simple data extraction to complex automated testing.
Basic Concepts of Selenium for Scraping
1. Understanding the WebDriver and Browser Control:
WebDriver acts as a bridge between your code and a web browser, allowing you to control browser actions programmatically. Each browser has its own WebDriver, which you can command to perform tasks like opening web pages, clicking buttons, or reading text, all through your script.
2. Navigating Pages and Handling Page Loads:
To navigate to a new page, you use methods like driver.get(“http://example.com”). Handling page loads in Selenium is crucial because you must ensure the page is fully loaded before attempting to extract any data.
Selenium can wait explicitly until certain conditions are met (like elements becoming visible) or implicitly wait for a specified timeout, helping avoid errors in your scrapes due to incomplete page loads. This ensures reliable data extraction even from dynamic web pages.
Accessing and Extracting Data
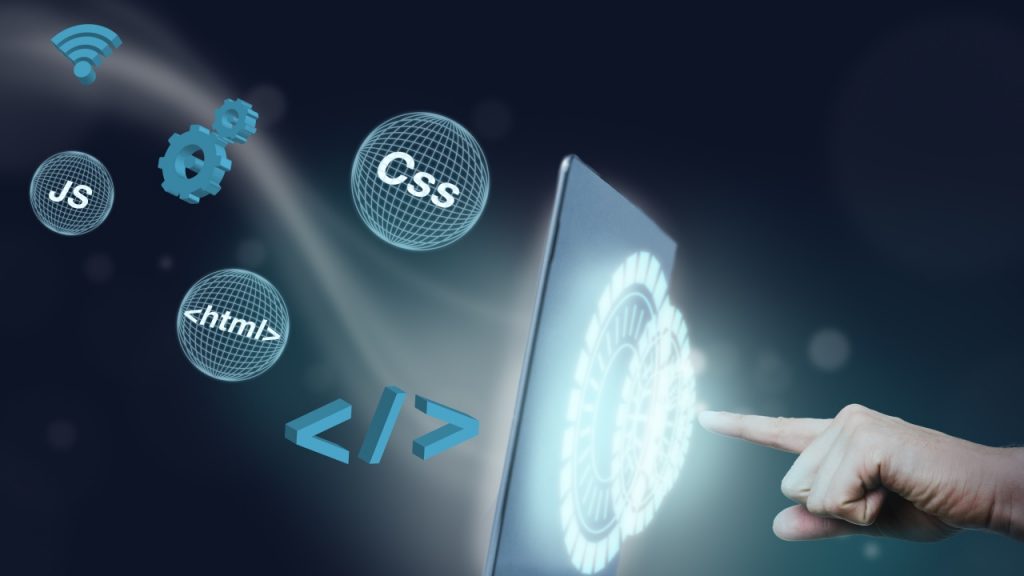
1. Techniques to Locate Elements (XPath, CSS Selectors):
Identifying the correct elements to scrape is key in web scraping. Selenium offers various methods to locate elements, including XPath and CSS selectors. XPath allows for navigation in the HTML structure based on elements’ attributes, relationships, or absolute paths.
CSS selectors provide a simpler way to select elements based on their style attributes. Learning to use these tools effectively can pinpoint the data you need quickly and accurately.
2. Extracting Text, Links, and Other Attributes from Elements:
Once elements are located, Selenium can retrieve the required data. Use the .text attribute to extract readable text and the .get_attribute(“href”) for links. For images, methods like .get_attribute(“src”) will fetch the image URLs. These techniques allow you to pull various data types from a page, enriching your dataset and providing a foundation for more complex data interaction scenarios.
Advanced Selenium Techniques
1. Handling AJAX and Dynamic Content:
AJAX and dynamically loaded content can pose challenges for web scraping. Selenium handles this by allowing you to wait for specific conditions using WebDriverWait and ExpectedConditions classes. This ensures that the page has loaded all elements before you attempt to scrape them, crucial for capturing data loaded in response to user actions or after initial page load.
2. Dealing with Pop-ups, Modals, and Notifications:
Pop-ups and modals often interrupt the scraping process. Selenium can interact with these elements by switching to the pop-up window, closing it, or extracting needed information from modals. It’s important to handle these gracefully to maintain the flow of automation.
3. Automating Login and Session Management:
For sites that require authentication, Selenium can automate the login process, entering usernames and passwords into form fields. Besides login, it can also manage session details, such as cookies, which are essential for maintaining access in a session-dependent scraping task. This capability is vital for scraping data across multiple pages that require a user to be logged in.
Practical Examples
1. Step-by-Step Guide to Scrape a Sample E-commerce Site:
- Initial Setup: Begin by configuring Selenium with the appropriate WebDriver for your browser. Ensure Selenium can interact with the browser by running a simple command to open a webpage.
- Navigating the Site: Use the driver.get(url) method to navigate to the e-commerce homepage.
- Locating Items: Employ CSS selectors or XPath to find product elements on the page. For example, locate a product list and iterate over items.
- Extracting Data: Use the .text method to pull product names and .get_attribute(“href”) for product links. To get prices, find the pricing element and extract its content.
- Handling Pagination: Implement loops to navigate through pagination elements. Detect the ‘next’ button and use Selenium to click it, then repeat the data extraction process on the new page.
2. Example of Scraping a Dynamic News Aggregator:
- Setting Up Event Listeners: Configure Selenium to wait for AJAX content to load using WebDriverWait. This ensures that dynamically loaded news articles are fully loaded before extraction.
- Data Extraction: Define precise selectors for news headlines and summaries. Extract these texts and any associated metadata, like timestamps or author names.
- Session Management: If access to certain content is restricted, use Selenium to automate login procedures by sending keys to login forms and handling any subsequent sessions or cookies.
- Continuous Scraping: Set a timer or trigger within your script to refresh the page content or navigate to newly updated sections periodically. Ensure your scraper can handle changes in site layout or content flow without breaking.
Challenges and Solutions in Web Scraping with Selenium
Knowing how to navigate the common challenges and their solutions will help you handle web scraping projects more effectively with Selenium. Here are a few of these discussed:
- Dynamic Content Loading: Elements that load asynchronously are often missed. Utilize Selenium’s WebDriverWait to ensure these elements are fully loaded before proceeding.
- Login Authentication and CAPTCHA Handling: Sites requiring login restrict access, and CAPTCHAs further complicate entry. Employ third-party CAPTCHA solving services or use rotating proxies to automate responses and mimic legitimate user behavior.
- Website Layout Changes and IP Bans: Frequent updates can disrupt scripts. Build flexible scripts with adaptable locators and use a range of rotating proxies to prevent IP bans, allowing continued access even when site designs change.
Conclusion
In conclusion, Selenium offers powerful capabilities for web scraping, allowing you to navigate complex web interactions with ease. As you refine your skills, keep exploring tools that enhance your scraping effectiveness. For a practical solution in extracting web content directly, check out URLtoText.com, an excellent resource for converting web pages into text, simplifying the process of data extraction.