The demand for data-driven decision-making has never been higher. Data drives decisions, and much of that data lives openly on the web. Instead of collecting it manually, you can use web scraping to extract information automatically and turn it into structured and usable content. From startups to enterprise-level organizations, businesses use scraped data to monitor trends, analyze competitors, and improve internal operations.
Knowing how to scrape the web gives you direct access to a wealth of raw data you can shape into actionable insights if you’re a data analyst, marketer, developer, or entrepreneur. This guide will help you understand how web scraping works, why it’s valuable, and how you can start building your own scrapers with the right tools and techniques.
Is Web Scraping Legal?
Before you start building web scrapers, it’s important to understand the legal and ethical considerations involved. While web scraping is widely used across industries, its legality depends on how and where you apply it.
Scraping publicly accessible data is generally legal, but that doesn’t mean it’s free of restrictions. Legal concerns often arise when scraping personal information, copyrighted content, or data behind login walls. In particular, commercial use of scraped data can introduce more risk. For a deeper look at these issues, check out our detailed article: Is Web Scraping for Commercial Use Legal?
Ethical vs. Unethical Scraping
Ethical scraping means respecting the boundaries of the website. This includes following robots.txt rules, using reasonable request rates, and avoiding any attempt to access restricted or personal information. Unethical practices include scraping sensitive data or overloading a server with requests. This can damage both your reputation and legal standing.
Fair Use and Terms of Service
Many websites allow data extraction for personal or academic use under fair use policies, but this varies case by case. Always read a site’s terms of service carefully, and when possible, use an official API to avoid legal complications. If you’re deciding between scraping a site or using its API, it’s worth reviewing our comparison on API vs. Web Scraping to understand the trade-offs.
Prerequisites to Learn Web Scraping
It helps to build a strong foundation in a few key areas before you start scraping websites. While you don’t need to be an expert, having a basic understanding of web technologies and programming concepts will make your learning process much smoother.
HTML & CSS (Understanding the DOM)
Web pages are built using HTML and styled with CSS. When you scrape a website, you’re essentially navigating its underlying structure—also known as the Document Object Model (DOM).
Understanding how HTML elements like <div>, <p>, <table>, and <a> are arranged will help you target and extract the data you need. You should be comfortable using browser developer tools to inspect and locate elements on a page. We have a guide on HTML to Plain Text Conversion to work with raw page content.
Programming Fundamentals (Python Recommended)
While web scraping is possible in several programming languages, Python is the most popular choice due to its readability and the wide range of scraping libraries available. You should understand basic programming concepts such as loops, functions, conditionals, and working with data structures like lists and dictionaries. If you’re new to Python, consider starting with beginner tutorials before moving on to web scraping libraries like BeautifulSoup, requests, or Scrapy.
HTTP Requests and APIs
To fetch data from a website, your scraper sends an HTTP request and receives a response—usually in the form of HTML. Understanding how HTTP works, including GET and POST requests, headers, status codes, and cookies, will help you interact with websites more effectively. It’s also useful to know the basics of APIs, since some websites provide structured data access through APIs as an alternative to scraping.
Best Programming Languages for Web Scraping
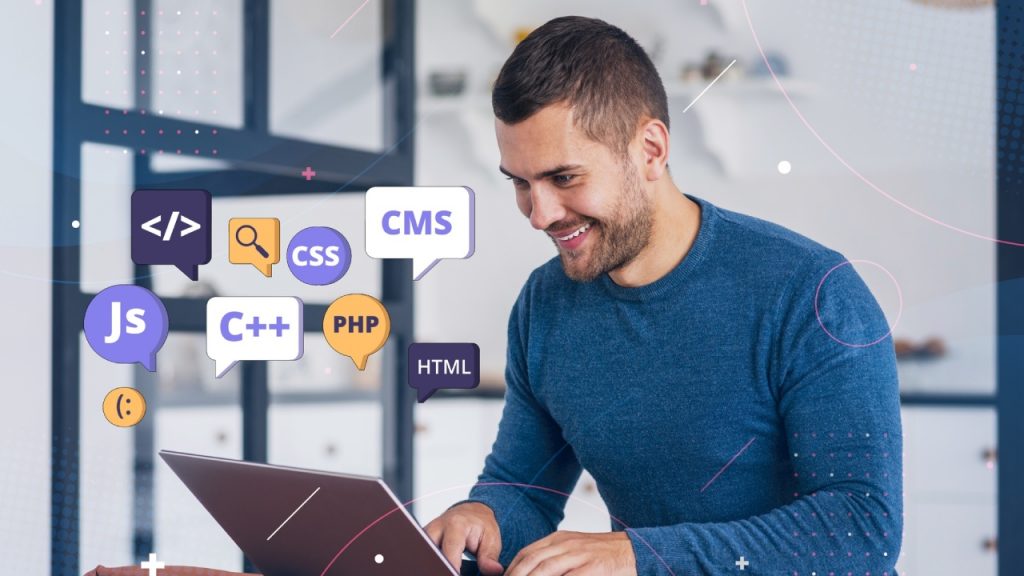
You can perform web scraping with several programming languages, but the best choice depends on your goals, the complexity of the target websites, and your existing skill set. Here are the top languages commonly used for scraping, along with their most popular tools and ideal use cases.
Python
Python is by far the most popular language for web scraping. Its clean syntax and powerful libraries make it beginner-friendly and efficient for handling web data. Three commonly used libraries include:
- requests: Used to send HTTP requests and receive responses from web pages.
- BeautifulSoup: A parsing library that helps extract specific data from HTML and XML.
- Scrapy: A more advanced framework that supports large-scale, fast, and flexible scraping projects.
When to use Python: Choose Python if you’re just starting out, building a small to medium-sized scraper, or working with mostly static websites.
JavaScript (Node.js)
JavaScript is ideal for interacting with dynamic and JavaScript-heavy websites. It can execute scripts in-browser, making it suitable for pages that rely on client-side rendering. Two popular tools include:
- Puppeteer: A headless browser that lets you control Chrome or Chromium to simulate real user interactions.
- Cheerio: A fast, lightweight HTML parser that mimics jQuery for server-side DOM manipulation.
When to use JavaScript: Use JavaScript if you’re scraping dynamic content, need to interact with front-end elements, or are already comfortable with Node.js.
Java
Java is a strong option for more structured and enterprise-grade scraping tasks. It offers robust performance and stability, especially for large or long-running scraping jobs.
- Jsoup: A powerful library for parsing and extracting data from HTML. It allows you to work with real-world HTML content in a straightforward way.
When to use Java: Consider Java if you’re building a scalable scraper for enterprise use or integrating scraping into a larger Java-based application. Check out our step-by-step guide on how to do web scraping in Java for a deeper dive into tools and code examples.
Key Tools and Libraries
To build an efficient and reliable web scraper, you’ll need the right tools. These tools and libraries will help you parse, inspect, and automate your scraping workflow.
Python Libraries
Python offers one of the most complete ecosystems for web scraping. Here are some of the most widely used libraries:
- requests: This library lets you send HTTP requests and retrieve the content of web pages. It’s lightweight and ideal for scraping static pages quickly and easily.
- BeautifulSoup: A powerful parsing library used to navigate and extract data from HTML or XML. It works well with requests to cleanly pull out elements like text, tables, or links.
- Scrapy: A full-featured scraping framework designed for large-scale or complex projects. It supports advanced features like request scheduling, middleware integration, and data export.
- Selenium: If a site loads data dynamically with JavaScript, Selenium can automate a real browser session to render the content before scraping. It simulates user actions such as clicking buttons or scrolling pages. If you want to master its use for scraping, check out our Selenium web scraping guide.
Each of these tools plays a different role. For basic scraping, start with requests and BeautifulSoup. For dynamic or large-scale jobs, consider using Selenium or Scrapy.
Chrome DevTools
Chrome DevTools is an essential tool for inspecting the structure of a webpage. By right-clicking an element and selecting “Inspect,” you can view its HTML tags, classes, and hierarchy. This helps you understand how to target the data you want with your scraper. You can also use the “Network” tab to monitor API calls or page load behavior.
Browser Extensions
Browser extensions can speed up the process of selecting elements on a page. One popular option is SelectorGadget, a Chrome extension that lets you click on elements to automatically generate the right CSS selector. This is especially helpful when you’re trying to identify the exact part of the page you want to extract.
Step-by-Step: How to Start Web Scraping
Getting started with web scraping can feel overwhelming, but breaking it down into manageable steps makes the process much easier. Here’s a simple workflow you can follow to build your first scraper.
1. Choose a Simple Target Website
Start by selecting a website with a clean structure and accessible data. Ideally, choose a site that allows scraping or provides open data. Public directories, product listings, blog archives, and news websites are good starting points. Make sure to check the site’s robots.txt file or terms of service to confirm scraping is permitted.
2. Inspect the Page Structure
Open the site in your browser and use Chrome DevTools (right-click > Inspect) to examine the HTML structure. Identify the specific elements that contain the data you want, such as product names, prices, article titles, or links. Look for patterns in the tags and classes so you can target them precisely.
3. Send a Request and Fetch the Page
Using a library like requests in Python, you can send an HTTP GET request to retrieve the page’s HTML content. This step downloads the source code that your scraper will analyze.
import requests
url = 'https://example.com/products'
response = requests.get(url)
html = response.text
4. Parse HTML and Extract Data
Use BeautifulSoup or a similar parser to navigate the HTML and extract the specific data points you’re targeting.
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('h2', class_='product-title')
for title in titles:
print(title.text)
This example looks for product titles within <h2> tags that have a specific class. You can adjust the tag and class names based on your inspection in Step 2.
5. Store or Export the Data
Once you’ve extracted the data, you can store it in a format that suits your needs. Common formats include:
- CSV: Easy to view and work with in Excel or Google Sheets.
- JSON: Useful for structured data and further processing.
- Databases: Ideal for large-scale scraping projects.
Here’s how to save data to a CSV file using Python:
import csv
with open('products.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Title'])
for title in titles:
writer.writerow([title.text])
Following these steps will help you build a basic scraper and understand the core workflow. As you gain confidence, you can expand your scraper to handle pagination, logins, and even dynamic content using tools like Selenium.
Common Challenges and How to Overcome Them
While web scraping is a powerful tool, it’s not always straightforward. Websites often use technologies or defenses that can make data extraction more complex. Here are some of the most common challenges you’ll face and how to deal with them.
Dealing with JavaScript-Heavy Websites
Some websites load content dynamically using JavaScript, meaning the data you want may not be present in the initial HTML response. In these cases, tools like requests and BeautifulSoup won’t work on their own.
How to overcome it:
- Use Selenium to render JavaScript in a real browser environment and access the fully loaded content. This allows you to simulate clicks, scrolling, and form submissions.
- Alternatively, inspect the Network tab in your browser’s DevTools to see if the data loads through a separate API request. If so, you can directly query that API, which is usually faster and cleaner.
Handling Pagination and Login Forms
Many websites split their content across multiple pages or require users to log in before viewing certain data.
How to overcome it:
- For pagination, identify the pattern in the URLs or use the “Next” button’s HTML to automate navigation through pages.
- For login forms, use requests.Session() in Python to maintain cookies and simulate login behavior. For JavaScript-based logins, use Selenium to interact with the login form like a real user.
Example of handling login with requests:
session = requests.Session()
payload = {'username': 'your_username', 'password': 'your_password'}
session.post('https://example.com/login', data=payload)
Detecting and Respecting Anti-Scraping Mechanisms
Websites may employ anti-scraping measures like rate-limiting, IP blocking, or CAPTCHAs to prevent automated access.
How to overcome it:
- Respect robots.txt to avoid scraping disallowed sections of the site.
- Throttle your requests by adding time delays between requests (time.sleep(1-3 seconds)).
- Rotate user agents and IP addresses using tools like proxies or libraries such as fake_useragent.
- Avoid scraping personal or sensitive information, and never overload a server with too many requests in a short time.
- For CAPTCHAs, consider using services or tools that solve CAPTCHAs, but only when legally and ethically appropriate.
Conclusion
Web scraping is a valuable skill that opens up countless opportunities to collect and analyze data from the web. By understanding the fundamentals, choosing the right tools, and following ethical practices, you can build powerful scrapers that serve a wide range of personal and professional goals.
Start small, practice often, and always respect the rules of the websites you interact with. The more you build, the more confident and capable you’ll become.