Web scraping is an essential technique for extracting valuable data from websites, enabling developers to gather information for analysis, research, or automation. With the rise of dynamic, JavaScript-heavy websites, traditional scraping methods often fall short.
JavaScript, however, provides powerful tools like Puppeteer and Cheerio, making it the go-to solution for scraping modern web pages that rely on client-side rendering. In this article, we’ll walk you through how to effectively scrape websites using JavaScript, covering the tools and libraries you’ll need, as well as key techniques for handling both static and dynamic content.
Setting Up for Web Scraping Using JavaScript
To begin scraping websites with JavaScript, you’ll need to set up a proper environment and understand the tools and libraries that can simplify the process. Below, we’ll go over the essential tools, prerequisites, and installation steps to get you started.
Tools and Libraries for JavaScript Web Scraping
Axios:
A promise-based HTTP client for making requests to websites. It’s commonly used to fetch HTML data from a webpage, which can then be parsed or processed further. Axios is a lightweight option that works well for static websites.
Cheerio:
A fast, flexible, and lean implementation of jQuery for the server, making it easy to parse HTML and navigate the DOM. It’s typically used in combination with Axios to extract specific data from static pages.
Puppeteer:
A powerful library for controlling headless browsers, such as Chrome or Chromium. Puppeteer is perfect for scraping dynamic websites that rely on JavaScript to render content. It allows you to automate browser actions like clicking buttons and scrolling, ensuring you capture the fully rendered content.
Playwright:
Similar to Puppeteer, Playwright is a newer library that supports multiple browsers (Chromium, Firefox, and WebKit) and provides a more robust solution for scraping complex websites. It offers more flexibility, especially when dealing with multi-browser support and advanced features.
Prerequisites for Setting Up a Scraping Environment
Before diving into scraping, make sure you have the following prerequisites:
- Node.js: JavaScript is primarily run on the server side for web scraping, and Node.js allows you to execute JavaScript code outside the browser. You can download and install Node.js from nodejs.org.
- NPM (Node Package Manager): NPM comes bundled with Node.js and is essential for managing libraries and dependencies needed for your project.
Installing Necessary Libraries and Dependencies
Once you’ve set up Node.js and NPM, you’ll need to install the required libraries. Follow these steps:
1. Create a New Project Directory:
Open a terminal or command prompt and create a new folder for your project.
mkdir my-web-scraper
cd my-web-scraper
2. Initialize a New Node.js Project:
Run the following command to create a package.json file, which will track your project’s dependencies.
npm init -y
3. Install Required Libraries:
Depending on your scraping needs, you can install Axios, Cheerio, Puppeteer, or Playwright. Here’s how you can install them:
npm install axios cheerio puppeteer
Or, if you want to use Playwright:
npm install playwright
4. Verify Installation:
After installation, check your package.json file to ensure the dependencies have been added correctly. You can also run the following command to confirm the libraries are installed:
npm list
Once these tools and libraries are installed, you’re ready to start building your web scraper in JavaScript. These libraries provide the foundation for making HTTP requests, parsing HTML, and automating browser interactions to extract the data you need from a variety of websites.
Understanding the Web Scraping Process with JavaScript
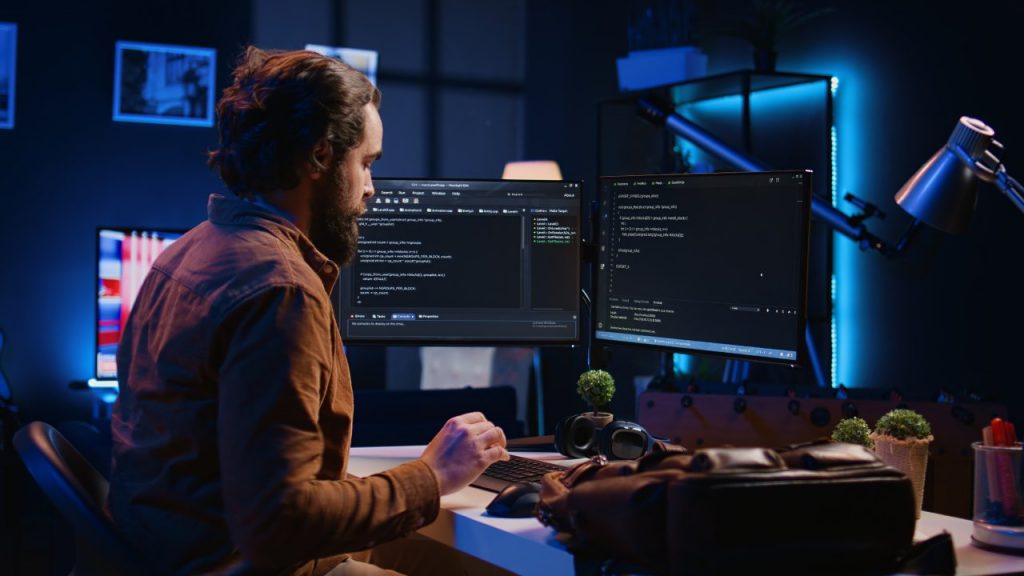
Web scraping involves several key steps to collect, parse, and extract useful data from websites. Below, we’ll break down the typical workflow involved in web scraping using JavaScript:
- Making an HTTP Request: The first step is to request the webpage content you want to scrape. This is done by sending an HTTP request to the website, which will return the HTML content of the page.
- Parsing the HTML Content: Once the page content is retrieved, you need to parse the HTML to extract the data you’re interested in. Parsing allows you to interact with the page’s structure programmatically.
- Navigating the DOM: The Document Object Model (DOM) represents the structure of the webpage. After parsing the HTML, you navigate the DOM to find and extract specific elements (e.g., titles, images, prices).
Extracting the Data: Finally, once you’ve located the necessary DOM elements, you can extract the desired data, which can be saved in a structured format (e.g., JSON, CSV) for further use.
Making HTTP Requests to Fetch Page Content (e.g., Using Axios)
To begin scraping, the first task is fetching the page content using HTTP requests. One of the most popular libraries for making HTTP requests in JavaScript is Axios.
Here’s how you can use Axios to fetch HTML content:
const axios = require('axios');
axios.get('https://example.com')
.then(response => {
console.log(response.data); // HTML content of the page
})
.catch(error => {
console.log('Error fetching the page:', error);
});
The axios.get() method sends a GET request to the specified URL and returns a promise with the page’s HTML content in response.data. Once you have this content, you can move on to parsing it.
Parsing HTML Content (Cheerio or Puppeteer)
After fetching the page content, the next step is parsing the HTML to extract the information you need. There are two popular approaches depending on whether you’re dealing with static or dynamic pages:
- Cheerio: If you’re scraping static HTML (pages where content is already rendered when the page loads), Cheerio is a great option. It provides a jQuery-like syntax for traversing the HTML DOM.
Here’s an example using Axios and Cheerio to scrape article titles from a webpage:
const cheerio = require('cheerio');
const axios = require('axios');
axios.get('https://example.com/articles')
.then(response => {
const $ = cheerio.load(response.data); // Load the HTML
$('h2.article-title').each((index, element) => {
console.log($(element).text()); // Extract and print article titles
});
})
.catch(error => {
console.log('Error:', error);
});
In this example, Cheerio loads the HTML content, and then we use the $(‘h2.article-title’) selector to target all the article titles, extracting their text with .text().
- Puppeteer: For scraping dynamic websites that rely on JavaScript for rendering content, Puppeteer is a better choice. It allows you to control a headless browser, meaning it can fully render JavaScript-heavy pages before scraping the data.
Here’s an example using Puppeteer to scrape content from a dynamic page:
const puppeteer = require('puppeteer');
async function scrapeData() {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/articles');
const titles = await page.evaluate(() => {
const titleElements = document.querySelectorAll('h2.article-title');
return Array.from(titleElements).map(element => element.textContent);
});
console.log(titles);
await browser.close();
}
scrapeData();
In this example, Puppeteer navigates to the page and uses the page.evaluate() method to extract the article titles from the fully rendered page.
Navigating the DOM and Extracting Data
Once you’ve parsed the HTML content, the next step is navigating the DOM to locate and extract the desired elements. Both Cheerio and Puppeteer provide simple ways to select elements within the DOM:
- Cheerio: Use familiar CSS selectors (e.g., $(‘div.classname’), $(‘ul > li’)) to find elements within the HTML structure.
- Puppeteer: The page.evaluate() method allows you to execute JavaScript within the browser’s context, enabling you to access the DOM as you would in a regular browser.
For example, if you’re scraping product details from an e-commerce site, you can navigate to the product name, price, and description using selectors, then extract this data:
const puppeteer = require('puppeteer');
async function scrapeProductData() {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/product/123');
const product = await page.evaluate(() => {
const name = document.querySelector('h1.product-name').textContent;
const price = document.querySelector('.product-price').textContent;
const description = document.querySelector('.product-description').textContent;
return { name, price, description };
});
console.log(product);
await browser.close();
}
scrapeProductData();
In this example, you’re navigating the DOM using CSS selectors to extract the product name, price, and description from a product page.
Best Practices for Web Scraping with JavaScript
Web scraping with JavaScript requires following best practices to ensure efficiency and minimize the risk of being blocked by websites or violating ethical and legal guidelines.Here are some specific best practices to keep in mind when scraping websites using JavaScript.
1. Respect robots.txt and Website Terms of Service
Before scraping any website, it’s crucial to check the website’s robots.txt file and terms of service. The robots.txt file specifies the areas of the website that are off-limits for crawlers, and scraping content that is disallowed can result in legal consequences or your IP being banned.
For example, you can check the robots.txt file of a site by going to https://example.com/robots.txt. If it’s restricted, you should avoid scraping those sections of the site.
// Example of checking robots.txt manually before scraping
axios.get('https://example.com/robots.txt')
.then(response => {
console.log(response.data); // Inspect the file for allowed or disallowed paths
})
.catch(error => console.error('Error reading robots.txt:', error));
2. Limit Request Frequency (Rate Limiting)
Excessive scraping requests can overwhelm a website’s servers, causing downtime or making your IP address appear suspicious. This is why it’s essential to implement rate limiting in your scraping scripts. By introducing delays between requests, you prevent overloading the server and reduce the risk of your IP being blocked. Use the setTimeout function in JavaScript to delay requests:
const axios = require('axios');
// Delay function
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function scrapeData() {
const urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3'];
for (const url of urls) {
try {
const response = await axios.get(url);
console.log(response.data); // Handle the response
await delay(2000); // Wait 2 seconds before making the next request
} catch (error) {
console.error('Error fetching data:', error);
}
}
}
scrapeData();
In this example, we fetch data from multiple pages, adding a 2-second delay between each request. This ensures that the server is not overwhelmed by rapid requests.
3. Handle Dynamic Content and Infinite Scrolling
Many modern websites load data dynamically as users scroll or interact with elements. If you’re scraping such a website, using libraries like Puppeteer or Playwright is critical since they allow you to automate the scrolling and capture content that is loaded dynamically. Simply sending a request for the initial HTML won’t work for dynamic sites.
For infinite scrolling sites, you can automate the scrolling process with Puppeteer:
const puppeteer = require('puppeteer');
async function scrapeInfiniteScroll() {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/infinite-scroll');
// Scroll the page 3 times
for (let i = 0; i < 3; i++) {
await page.evaluate(() => {
window.scrollBy(0, window.innerHeight); // Scroll down by one viewport
});
await page.waitForTimeout(2000); // Wait 2 seconds for content to load
}
// Extract data
const data = await page.evaluate(() => {
const items = [];
document.querySelectorAll('.item').forEach(item => {
items.push(item.textContent);
});
return items;
});
console.log(data);
await browser.close();
}
scrapeInfiniteScroll();
Here, the script scrolls down the page multiple times, waits for content to load, and then extracts the data, ensuring it captures all dynamically loaded items.
4. Use Proxies and Rotate User Agents
Websites often implement measures to detect and block scrapers based on their IP addresses or user-agent strings. To avoid detection, using proxies and rotating user-agent strings can help disguise your scraping efforts.
Proxies:
Proxies allow you to route your requests through different IP addresses, making it harder for websites to track and block you. You can use proxy services like ScraperAPI, ProxyMesh, or residential proxies to rotate your IP address.
User-Agent Rotation:
Rotating user-agent strings can prevent websites from detecting that requests are coming from a bot. Here’s an example of rotating user agents in Axios:
const axios = require('axios');
const userAgents = [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_0) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/79.0.3945.88 Safari/537.36',
'Mozilla/5.0 (Linux; Android 10; Pixel 3 XL Build/QP1A.190711.020) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Mobile Safari/537.36'
];
function getRandomUserAgent() {
return userAgents[Math.floor(Math.random() * userAgents.length)];
}
axios.get('https://example.com', {
headers: {
'User-Agent': getRandomUserAgent()
}
})
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
This code randomly selects a user-agent string from an array for each request, making it harder for the website to identify patterns indicative of scraping.
5. Handle Errors and Retry Logic
Web scraping often involves network instability, server issues, or even temporary blocks. Implementing error handling and retry logic can improve the reliability of your scraper. For example, if a request fails, you can retry the request a few times before giving up.
Here’s an example of implementing retry logic with Axios:
const axios = require('axios');
async function fetchData(url, retries = 3) {
try {
const response = await axios.get(url);
return response.data;
} catch (error) {
if (retries > 0) {
console.log(`Retrying... (${retries} attempts left)`);
return fetchData(url, retries - 1); // Retry the request
} else {
console.error('Failed after multiple retries:', error);
}
}
}
fetchData('https://example.com')
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This script retries the request up to three times before failing. It’s an essential practice for handling network errors or temporary server issues.
Conclusion
Web scraping with JavaScript offers a practical way to collect and process data from websites. Whether you’re dealing with static content using tools like Axios and Cheerio, or dynamic pages with Puppeteer, JavaScript provides the flexibility needed for efficient data extraction.
By following the best practices mentioned, you can ensure your scraping projects run smoothly while staying respectful of the websites you’re accessing. For more insights on selecting the right tools for your scraping needs, check out this article on the best web scraping tools. With the right tools and approach, you can effectively harness web data for a variety of needs.