Web scraping is the process of extracting data from websites and converting it into a structured format, such as CSV or JSON, for easy analysis. This technique is widely used for tasks like market research, price comparison, and content aggregation. By automating the extraction of large volumes of data, web scraping saves time and resources compared to manual collection.
Java is a powerful and reliable programming language for web scraping. It offers a range of libraries like JSoup, Selenium, and HtmlUnit. These tools allow developers to handle both simple and complex scraping tasks efficiently, with Java’s performance and stability ensuring the process runs smoothly, even with large datasets. This guide will walk you through how to scrape websites using Java, from setting up your environment to working with dynamic content.
Why Use Java for Web Scraping?
Java is a powerful and widely-used programming language that excels in web scraping for several key reasons. Below are some of the main reasons why Java stands out for web scraping tasks:
Java’s Strength in Handling Large Datasets
One of Java’s key advantages is its ability to handle large volumes of data efficiently. Web scraping often involves working with extensive datasets from multiple websites, and Java is well-suited for processing this data without compromising performance.
Its built-in support for multithreading allows developers to scrape multiple web pages in parallel, speeding up the overall process. Java’s memory management and garbage collection systems also ensure that even large datasets are processed efficiently without overwhelming system resources.
Java’s Rich Ecosystem of Libraries and Tools
Java boasts a wide variety of libraries and frameworks specifically designed to make web scraping easier and more effective. Popular tools like JSoup, Selenium, and HtmlUnit offer powerful features to parse HTML, interact with dynamic content, and simulate browser actions.
These libraries are well-documented and widely used, ensuring that developers have access to community support and continuous updates. The flexibility and ease of integration of these tools make Java a versatile choice for web scraping projects of all sizes.
Cross-Platform Compatibility and Performance
Java is known for its cross-platform capabilities, meaning that web scraping scripts can run on different operating systems without modification. Whether you’re working on Windows, macOS, or Linux, Java ensures that your scraping code is portable and consistent. This is particularly useful in collaborative or production environments where different team members may use different operating systems.
Additionally, Java’s high performance and scalability make it ideal for both small and large-scale scraping operations, ensuring that even complex scraping tasks can be handled quickly and reliably.
Essential Java Libraries for Web Scraping
Several Java libraries make the web scraping process easier and more efficient. Each library has its unique strengths, and depending on the type of data you want to scrape and the structure of the website, you may choose one or a combination of them. Below are some of the most essential libraries for web scraping in Java:
JSoup:
JSoup is a popular Java library used for parsing and extracting data from HTML. It simplifies web scraping by providing a simple API for navigating the HTML structure and extracting elements like text, attributes, and links.
Features:
- Parses HTML from URLs, files, or strings.
- Supports CSS selectors for selecting elements.
- Allows easy manipulation of HTML elements (adding/removing content).
- Robust error handling for dealing with malformed HTML.
- Clean and easy-to-read syntax for extracting data.
Example Code for Parsing HTML:
java
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
public class JsoupExample {
public static void main(String[] args) {
try {
// Connect to the website and parse its HTML
Document doc = Jsoup.connect(“https://example.com”).get();
// Select all hyperlinks using CSS selectors
Elements links = doc.select(“a[href]”);
for (Element link : links) {
System.out.println(“Link: ” + link.attr(“abs:href”));
System.out.println(“Text: ” + link.text());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
This snippet demonstrates how JSoup can fetch a webpage, parse its HTML, and extract all hyperlink URLs and their corresponding text.
HtmlUnit
HtmlUnit is a headless browser that emulates a web browser’s behavior without a graphical user interface. It is especially useful for scraping content that relies on JavaScript.
Features & Use Cases:
- Simulates a real browser, complete with JavaScript and AJAX support.
- Ideal for testing, navigating, and interacting with web pages programmatically.
- Useful for scraping sites that require form submission or user interaction
How to Use HtmlUnit:
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
public class HtmlUnitExample {
public static void main(String[] args) {
try (final WebClient webClient = new WebClient()) {
// Optimize performance by disabling unnecessary features
webClient.getOptions().setCssEnabled(false);
webClient.getOptions().setJavaScriptEnabled(true);
// Fetch and process the web page
HtmlPage page = webClient.getPage(“https://example.com”);
System.out.println(page.asText());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, HtmlUnit is used to load a page, process any JavaScript, and output the page’s textual content.
Selenium
Selenium is well-known for automating web browsers and is ideal for scraping dynamic content. Let’s discuss when to use Selenium:
- Dynamic Content Handling: When dealing with pages that rely heavily on JavaScript for rendering content.
- User Interaction Simulation: For navigating through pages, clicking buttons, or filling out forms.
- Testing and Scraping Integration: Provides a real browser context which is particularly helpful for complex scraping tasks.
For scenarios requiring authentic browser interactions, Selenium automates a full browser (e.g., Chrome or Firefox), ensuring that dynamic elements are fully loaded before data extraction begins.
Apache HttpClient
Apache HttpClient is a robust library designed for handling HTTP requests and responses. Here are key features:
- Supports all standard HTTP methods (GET, POST, PUT, DELETE, etc.).
- Offers granular control over HTTP connections, headers, and cookies.
- Ideal for low-level HTTP operations, such as handling redirects or interacting with RESTful APIs.
It’s often used to perform direct HTTP operations where libraries like JSoup are either not needed or can be combined for more specialized tasks.
Jsoup and Selenium Combination
Combining JSoup with Selenium leverages the strengths of both libraries, making your scraping workflow more efficient. The combination leads to improved efficiency:
- Dynamic Content Handling: Use Selenium to load pages and render JavaScript, ensuring that dynamic content is fully loaded.
- Efficient Parsing: Once the page is rendered, pass the HTML source to JSoup for fast and flexible parsing.
Example Workflow
java
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class JsoupSeleniumExample {
public static void main(String[] args) {
// Configure the ChromeDriver path
System.setProperty(“webdriver.chrome.driver”, “/path/to/chromedriver”);
WebDriver driver = new ChromeDriver();
try {
// Use Selenium to navigate to the target page
driver.get(“https://example.com”);
// Optional: Wait for dynamic content to load
Thread.sleep(5000);
// Get the page source once fully loaded
String pageSource = driver.getPageSource();
// Parse the rendered HTML using JSoup
Document doc = Jsoup.parse(pageSource);
System.out.println(“Page Title: ” + doc.title());
} catch (Exception e) {
e.printStackTrace();
} finally {
driver.quit();
}
}
}
Here, Selenium ensures that all dynamic content is loaded, and JSoup handles efficient data extraction from the fully rendered HTML.
Setting Up a Java Development Environment for Web Scraping
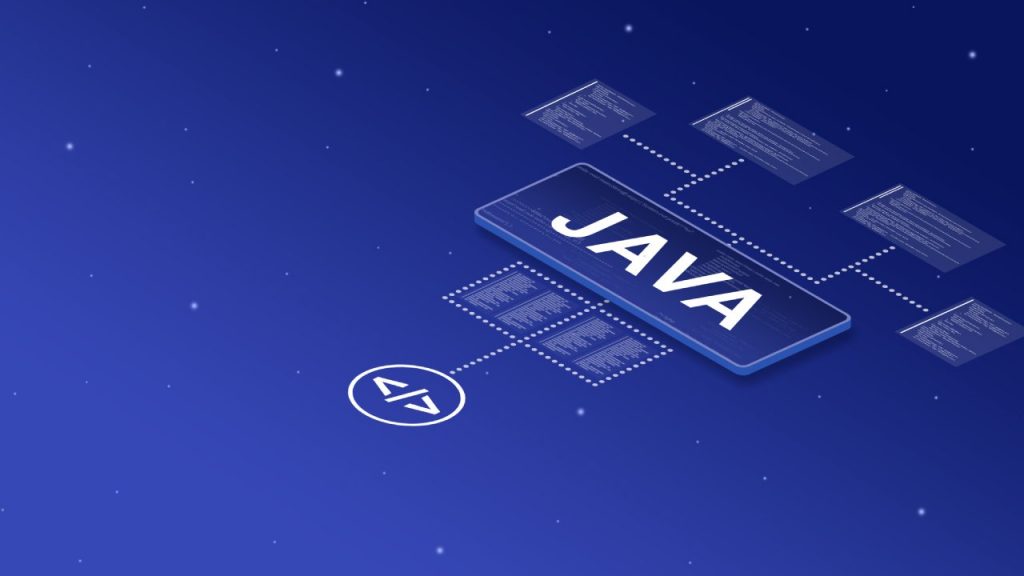
To get started with web scraping in Java, you’ll need to set up a development environment with the necessary tools, dependencies, and libraries. Here’s a step-by-step guide on how to configure your environment for effective web scraping.
Install Java Development Kit (JDK):
Java is the primary programming language for web scraping in this guide. To begin, you’ll need to install the Java Development Kit (JDK). The JDK includes all the tools you need to compile and run Java programs. You can download the latest version of the JDK from the Oracle website or from AdoptOpenJDK.
After downloading and installing the JDK, ensure that the java and javac commands are available in your terminal or command prompt by adding the JDK’s bin directory to your system’s PATH variable.
Set Up an Integrated Development Environment (IDE):
An IDE will make writing and managing your web scraping code easier. Popular Java IDEs include:
- IntelliJ IDEA: A feature-rich IDE for Java development with excellent support for web scraping libraries.
- Eclipse: Another popular IDE with a strong Java community and extensive plugin support.
- NetBeans: Known for its user-friendly interface and robust Java support.
Download and install your chosen IDE. Once installed, you can create a new Java project for web scraping.
Setting Up Maven or Gradle Dependencies
Java projects often use build tools like Maven or Gradle to manage dependencies. These tools allow you to easily add libraries and packages needed for your project. In this case, you’ll need to import libraries like JSoup, HtmlUnit, and others for web scraping.
1. Maven:
Maven is a popular build tool for Java. To use Maven, you’ll need to create a pom.xml file in your project’s root directory.
Add the following dependencies to your pom.xml file for JSoup, HtmlUnit, and Selenium:
<dependencies>
<!– JSoup for HTML parsing –>
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>1.14.3</version>
</dependency>
<!– HtmlUnit for headless browser simulation –>
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit</artifactId>
<version>2.48.5</version>
</dependency>
<!– Selenium for dynamic content scraping –>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
</dependencies>
Once you’ve added these dependencies, Maven will automatically download the required libraries for you when you build the project.
2. Gradle:
Gradle is another powerful build tool for Java. To use Gradle, you’ll need to create a build.gradle file in your project’s root directory.Add the following dependencies to your build.gradle file:
dependencies {
// JSoup for HTML parsing
implementation ‘org.jsoup:jsoup:1.14.3’
// HtmlUnit for headless browser simulation
implementation ‘net.sourceforge.htmlunit:htmlunit:2.48.5’
// Selenium for dynamic content scraping
implementation ‘org.seleniumhq.selenium:selenium-java:4.0.0’
}
Gradle will fetch the necessary libraries when you sync your project.
Importing Required Libraries (JSoup, HtmlUnit, Selenium)
Now that your project is set up, you can start importing the libraries into your Java classes.
1. JSoup:
JSoup is typically used for parsing and extracting data from static HTML content. Once your project dependencies are set up, you can import JSoup as follows:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
2. HtmlUnit:
HtmlUnit is useful for simulating browser interactions with headless browsing. Import HtmlUnit like this:
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
3. Selenium:
Selenium is used for scraping dynamic web pages, especially those that rely on JavaScript. To use Selenium, import the necessary classes like this:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
Once the libraries are imported, you can begin writing Java code to scrape data from websites using these tools.
Testing Your Setup
After setting up everything, it’s a good idea to run a simple test to ensure that all the libraries are properly integrated.
For example, a basic JSoup test might look like this:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class WebScrapingTest {
public static void main(String[] args) {
try {
// Fetch and parse HTML from a website
Document doc = Jsoup.connect(“https://example.com”).get();
System.out.println(“Title: ” + doc.title());
} catch (Exception e) {
e.printStackTrace();
}
}
}
If the above code runs successfully, it confirms that JSoup is set up correctly and ready to be used for scraping.
Conclusion
Web scraping in Java is an essential skill for anyone looking to automate data extraction from websites. By following the steps and utilizing the right tools, beginners can quickly start building their own scraping applications.
For those looking to simplify the process of converting URLs to text or extracting content from webpages, tools like URL to Text can be incredibly helpful. With the right setup and knowledge, you can unlock the full potential of web scraping and leverage data for various projects and analyses.